This post is completed by 1 user
|
Add to List |
182. Binary Tree-Postorder Traversal - Non Recursive Approach
Objective: Given a binary tree, write a non-recursive or iterative algorithm for postorder traversal.
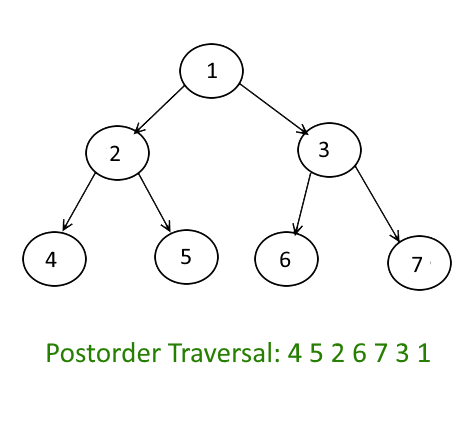
Example:
Earlier we have seen "What is postorder traversal and recursive algorithm for it", In this article, we will solve it in an iterative/Non Recursive manner.
Approach:
- We have seen how we do inorder and preorder traversals without recursion using Stack, But post-order traversal will be different and slightly more complex than the other two. The reason is post order is non-tail recursive ( The statements execute after the recursive call).
- If you just observe here, postorder traversal is just the reverse of preorder traversal (1 3 7 6 2 5 4 if we traverse the right node first and then the left node.)
- So the idea is to follow the same technique as preorder traversal and instead of printing it push it to another Stack so that they will come out in reverse order (LIFO).
- At the end just pop all the items from the second Stack and print it.
Pseudo Code:
Push root into Stack_One. while(Stack_One is not empty) Pop the node from Stack_One and push it into Stack_Two. Push the left and right child nodes of the popped node into Stack_One. End Loop Pop-out all the nodes from Stack_Two and print it.
See the animated image below and the code for more understanding.
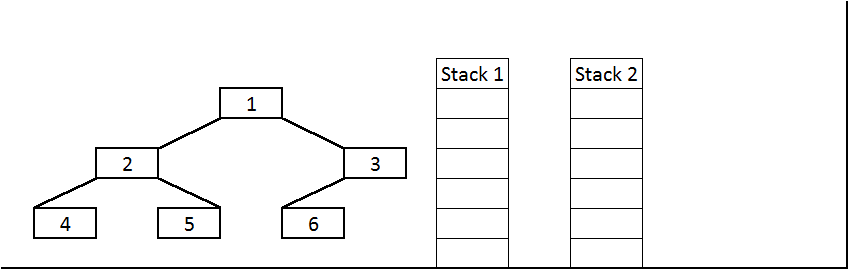
Output:
4 5 2 6 7 3 1 4 5 2 6 7 3 1