Be the first user to complete this post
|
Add to List |
389. Convert Roman Number to Integer
Objective: Given a Roman number, write a program to convert it to Integer.
Roman Number - Letters used in Roman numerals and the corresponding numerical values are given in the table below.
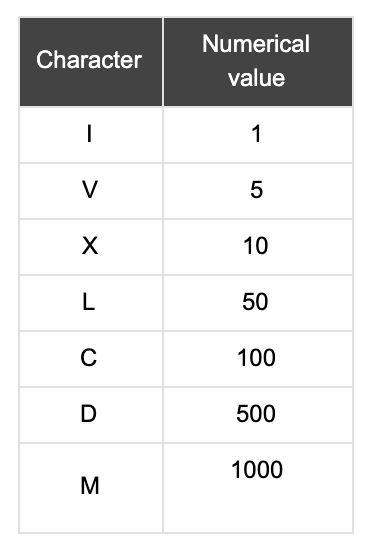
Rules:
Roman numerals are usually written in highest to lowest from left to right except for some special cases where left character is less than the right character. for example 'IV' is equivalent to 4 not 'IIII'. In such cases, subtract the left character value from the right character value. 'IV' will be 5-1 = 4, same for 'IX' = 10-1 = 9. Below are the cases -
- I can be placed before V or X, represents subtract one, so IV (5-1) = 4 and 9 is IX (10-1)=9.
- X can be placed before L or C represents subtract ten, so XL (50-10) = 40 and XC (100-10)=90.
- C placed before D or M represents subtract hundred, so CD (500-100)=400 and CM (1000-100)=900.
Example:
Roman: XXV Integer: 25 --------------------------------------------------- Roman: XXXVI Integer: 36 --------------------------------------------------- Roman: MXXIII Integer: 1023 --------------------------------------------------- Roman: DXLII Integer: 542
Approach:
- Iterate through given roman number from right to left (reverse).
- Initialize result = 0.
- Take one character at a time and check its corresponding numeral from the table and add it to the result.
- The only special case that needs to be considered is when the character at left has smaller value than the character at right. for example 'IX' for 9 or 'IV' for 4, in such cases subtract left character value from the result. Except for this special case, In all other cases, all the pairs have left character value >= right character value.
Walk Through:
values = [1000,900,500,400,100,90,50,40,10,9,5,4,1] String[] romanLiterals = ["M","CM","D","CD","C","XC","L","XL","X","IX","V","IV","I"]; Input: XCIX result =0 Iterate through right to left. Processing character: X result = 0 + 10 = 10 Processing character: I Compare it with the character at right - X I less than X, reduce the value of I from the result result = 10 - 1 = 9 Processing character: C Compare it with the character at right - I C greater than I, add the value of C to the result result = 100 + 9 = 109 Processing character: X Compare it with the character at right - C X less than C, reduce the value of X from the result result = 109 - 10 = 99
Output:
Roman Number: MIXX Integer: 1019 ------------------------------------ Roman Number: IXX Integer: 19 ------------------------------------ Roman Number: DXLII Integer: 542 ------------------------------------ Roman Number: MXXIII Integer: 1023 ------------------------------------